Connect Four
Goals
The purpose of this assignment is to learn to
- Use booleans to create a 2-player game.
- Use loops, 2D arrays, and conditional statements to drop pieces onto the grid and check if four of the same color have connected.
Programming
Tasks
- Initialize a 2D array the size of your game grid
- Determine which player will go first
- Create a function that uses keypress events to move the game piece around
- Create a function that handles player turns
- Create functions that check for the win condition
- Create a win condition
- Create a tie condition
- Correctly handle edge cases
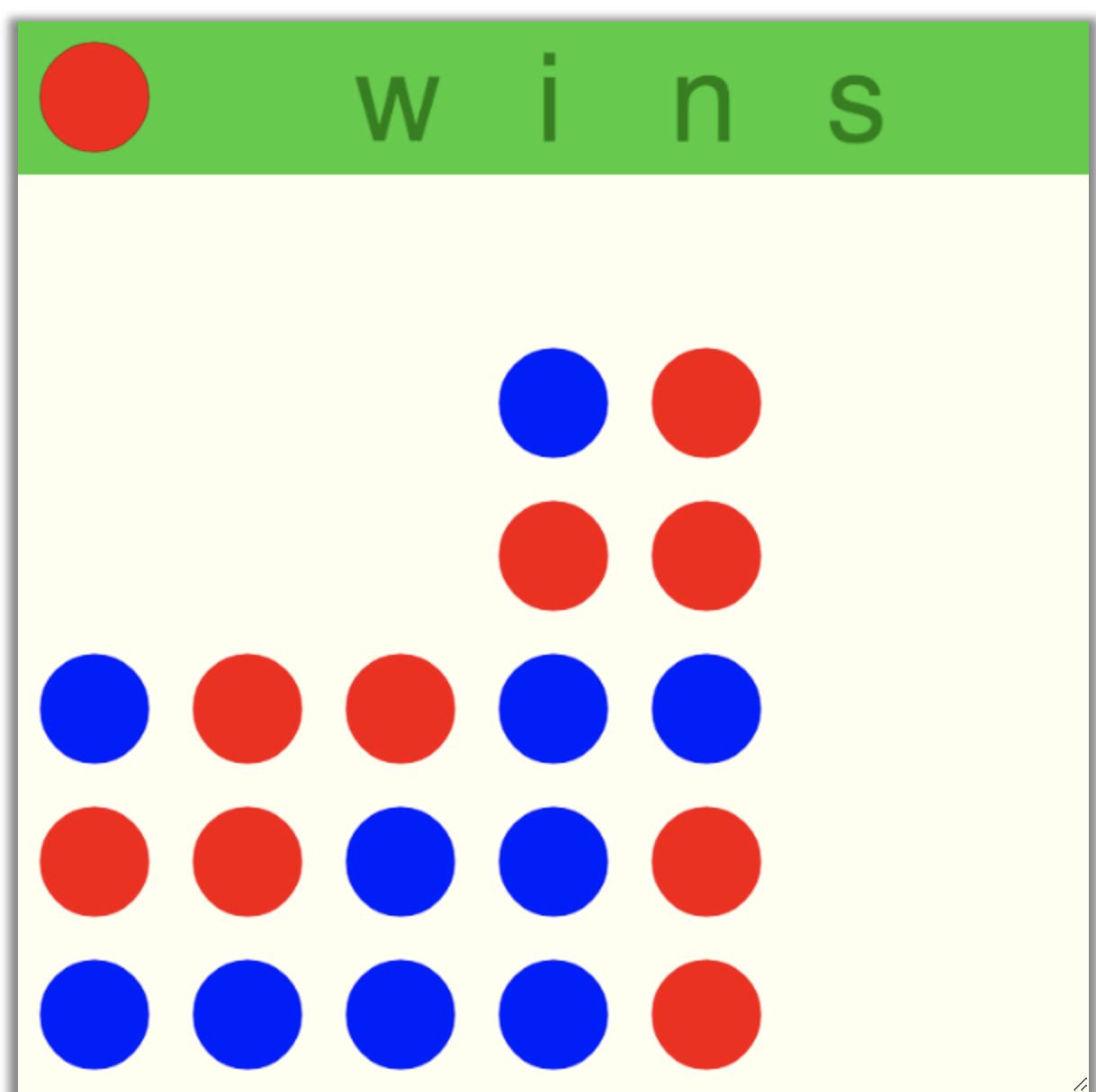
More Details to Get You Started
Key Press Events - NonBlocking Games
- keyUp()
- keyDown()
- keyLeft()
- keyRight()
Variables, Colors, and Sprite Symbols
- NamedSymbol.symbolname;
- NamedColor.colorname;
- drawSymbol(column, row, NamedSymbol, NamedColor);
- setBGColor(column, row, NamedColor);
Important Functions
- The gameLoop() function loops until the win condition has been met (or there is a tie).
- quit() stops the game
- start() starts the game and calls the initalize function once before starting the gameLoop()
Help
Java
NonBlocking Games Documentation
NamedColor Documentation
NamedSymbol Documentation
C++
NonBlocking Games Documentation
NamedColor Documentation
NamedSymbol Documentation
Python
NonBlocking Games Documentation
NamedColor Documentation
NamedSymbol Documentation