Singly Linked List Tutorial
SLelement<E> is the basic element used to implement a singly linked list in BRIDGES and is inherited from Element<E>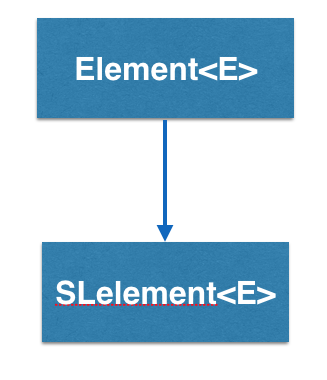
How does the SLelement<E> work?
SLelement<E> stands for Singly Linked Element and is a type
of container that has one link, pointing to another SLelement<E>.
So an SLelement<E> "knows" who it is pointing at but it does not
know who is pointing at it(if any). The generic parameter E can be any
application specific data type that the list can hold.
In the above example, SLelement1 points to SLelement2. Calling getNext()
on SLelement1 will return a link to SLelement2, and calling getNext() on
SLelement2 will return a link to SLelement3. SLelement3 is not pointing
to another SLelement so calling getNext() on SLelement3 will return NULL.
This is desirable since you will know that you have reached the end of the
singly linked list (as NULL is a unique value and not a legal memory address
for an object).
Also notice that there is no getPrev(). SLelement2 has no idea what
element came before it. So, you CANNOT go backwards.
This tutorial will illustrate the use of singly linked lists in BRIDGES. The tutorial consists of 3 parts:
- A basic tutorial on how to create a small singly linked list out of a few elements and visualize it
- How to style the list with visual attributes
- Advanced features, such as iterators and range loops that can be used with the list
See also
This tutorial gives an introduction to the usage of singly linked list. You can find the complete documentation of the features in the Doxygen documentation of the following classes and functions:
- SLelement [Java] [C++] [Python]
- Element [Java] [C++] [Python]
- ElementVisualizer [Java] [C++] [Python]
- LinkVisualizer [Java] [C++] [Python]
- Color [Java] [C++] [Python]
1. Getting Started: Build a Barebones Singly Linked List
In the first part of the tutorial, we will create a singly linked list with string labels, provide BRIDGES a handle to the data structure and visualize the list. Here is the code for this tutorial. The expected visualization follows that. Hit the 'l' button to turn on the labels.
Make sure that you can run the basic tutorial.
If you follow the URL given to you when the application runs, it will get to to the Bridges webpage that shows your output (also shown below). You do not need to be logged into your BRIDGES account to see the output. If you are logged into your account, the output will show up in your gallery.
2. What Visual Attributes are supported for Singly Linked Lists?
The list you created in the first part of the tutorial uses default attributes and is pretty boring, but it gives you the basic structure of a BRIDGES program.
Next, we will style the list we just created. For linked lists, you can set the shape, color, opacity and label of the elements, and for the links, color, opacity, thickness and label. Check out the links to the classes (listed above) that supports these attributes and also details the possible colors and shapes you can use and how to specify them.
The following code styles the linked list we created in part 1 and adds visual attributes. The expected visualization is below that.
3. Advanced Features.
In the last part of this tutorial, we show some advanced features with singly linked lists. This depends on the programming language, to some extent. For lists, we demonstrate traversal of the list by using a regular loop, through iterators and range loops. These features make it more intuitive and convenient to traverse the data structure.
The following code illustrates these advanced features of singly linked lists. The visualization is below that.
Well done! You’ve just created your Bridges Singly Linked List!
Going Further
Check Bridges assignment page for linked list based assignments